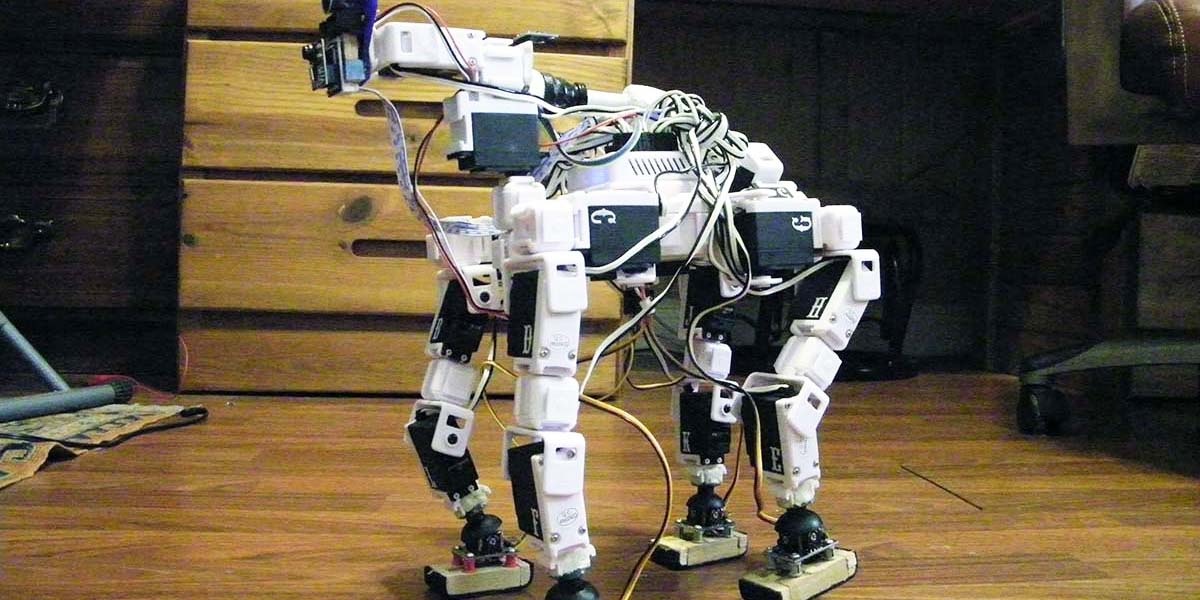
Experimenting with Walking Robots
By John Blankenship View In Digital Edition
The movements of hobbyist-oriented walking robots are often controlled by frame-based software that has severe limitations. This article discusses hardware and software options that could allow your robot to move more intelligently over uneven terrain by utilizing sensory data.
I’ve always been intrigued by walking robots, but when I started looking for a platform that suited my needs, I became a little discouraged. I liked the EZ-Robot hardware because its clip-together servos provide the flexibility to add or remove joints and limbs based on my current needs.
Figure 1 shows a four-legged (dog-like) walker I created from EZ-Robot parts and a few custom modifications.
FIGURE 1.
Leg Joints
Each leg has three joints, a hip, and a knee as you would expect, plus a shoulder for rotating the leg (for turning the robot). Figure 2 provides a close-up view of the leg. Notice the robot’s foot in this figure. It provides a natural-looking ankle flex while walking, but without having to utilize any motors.
FIGURE 2.
The main component in each foot is a cheap joystick assembly (see Figure 3). Originally, I wanted to use the X-Y pot readings to determine the ankle’s orientation, but the quality of the potentiometers made that unrealistic. I decided to use them anyway though, just because they made a great physical ankle.
FIGURE 3.
I wanted a way to determine when a foot was elevated (and when it contacted the floor), so I enclosed a snap-action leaf switch mounted in a base made from balsa wood. The bottom of each foot was eventually wrapped with foam material to improve traction.
Shifting the Robot’s Weight
When the robot wants to step with a particular leg, it needs to shift its weight away from that leg. It can do that by shortening appropriate legs, but my creature can use its tail to aid in this endeavor.
Notice in Figure 4 that the end of the tail has a relatively heavy steel pipe adapter (1/2 inch to 3/4 inch). As the robot swings its tail using one rotational and one lever servo, the weight of this piece can alter the robot’s center of gravity.
FIGURE 4.
Also notice in Figure 4 that the robot’s right front leg is swinging outward. Giving each leg this ability makes it possible for the robot to turn as it walks — or even turn in place.
Figure 5 shows the robot actually taking a step with its left rear leg. Notice how the right front leg is shortened to move the weight away from the stepping leg. Notice also that the tail is moved right and forward to help with the desired weight shift.
FIGURE 5.
The Head Sensors
Eventually, I want the robot to be able to avoid objects as it walks. I wanted as many options as possible, so I created a head composed of a variety of sensors as shown in Figure 6.
FIGURE 6.
The eyes are an SR04 ultrasonic sensor (directly supported by EZ-Robot). Above the eyes is an ultrasonic sensor with an analog output, which is actually easier for me to use (more on this later).
Below the eyes is an IR ranging sensor that also provides an analog output. The combination of ultrasonic and IR ranging can be very beneficial — especially with the head’s ability to move both left and right and up and down.
Finally, an EZ-Robot camera is mounted below the IR sensor. This array of sensors should provide hours of fun because the options for experimenting are nearly unlimited from a hobbyist’s perspective.
Adding an Accelerometer
Experimenting with the robot on sloping surfaces will require that the software be able to determine if the robot’s body is level, so I added an accelerometer. It’s shown in the close-up in Figure 7.
FIGURE 7.
For a positional reference, you can also see the accelerometer mounted just behind the head in Figures 1 and 4. I used an ADXL335 because it uses analog voltages to indicate the sensor’s angular orientation.
Software Considerations
As much as I like the EZ-Robot hardware’s flexibility, I was annoyed by the inflexibility of EZ’s software for moving a walking robot. To be fair, they — like most other producers of walking software for hobby robots — utilize a frame-based system.
Using an editor to build a variety of frames composed of joint positions and then transitioning between those frames can be a very quick (and often effective) way of creating a walking motion — especially for beginners.
It’s almost impossible, though, to create a robot that can handle uneven terrain with frame-based motion. Because of that, I wanted to find a way to control the robot’s servos in a much more flexible manner than I felt was possible with the EZ-Robot environment. With a little research, I found the EZ-B V4.x Datasheet and Communication Protocol. It provided the information I needed to send commands and receive sensory data from the EZ-Robot controller using almost any language capable of TCP communication.
Those not familiar with TCP communication could benefit from my article on controlling robots over the Internet here.
RobotBASIC
Once I understood the EZ protocol, I created subroutines with RobotBASIC (a free language available from (www.RobotBASIC.org) to move servomotors and to read digital and analog port data (which explains why I chose ranging sensors with analog outputs).
My next step was to create routines that could move servos not just to fixed positions, but to positions relative to their current positions. Let’s see why that’s important.
Assume we want the robot to step with one of its legs. It needs to raise the leg by bending both the hip and knee and then extending the leg forward to complete the step.
Normally, this would mean the leg extends fully in order to get the foot back on the ground.
When programming the robot to navigate over uneven or sloped terrain, however, the leg should only extend until the foot hits the ground (as determined by a foot sensor; see Figure 3). Later, we might need to shorten the stepped leg slightly to shift the robot’s weight in some way.
Since the leg is already shorter than normal, we need the ability to move the joints relative to their current positions (which were determined by the terrain when the leg was stepped).
Sometimes, of course, we might also need to move joints relative to their centered positions (that is to an absolute — not relative — position).
Because of this, the software needs to use arrays to keep track of the center positions and the current positions of every servomotor in the system.
A Servo-Center Editing Tool
The need for an array containing the center positions of all servos generated a need for editing software for creating that array. It should allow the user to position each servo on the robot to a centered or normal position.
These positions would be displayed as the servos are moved and stored in an array that can be saved and retrieved as needed.
Figure 8 shows a sample output from such an editing program written in RobotBASIC.
FIGURE 8.
The center-position editor itself is actually a routine that is callable from the main robot control program whose startup screen is shown in Figure 9.
FIGURE 9.
It also lets you initialize the LAN connection between RobotBASIC and the EZ controller, and run an application program (in this case, the routine that makes the robot walk).
Control Subroutines
The first step in creating a walking routine was to build routines that perform actions such as moving the tail, shortening a leg, initiating a step, moving the body forward, etc. A simple example of one such routine is shown in Figure 10.
sub BodyForward(Amount)
for j=2 to 11 step 3
call FromCurServoPos(j,-Amount)
next
return
FIGURE 10.
It uses a FOR loop to move all four hip joints backward by a specified amount (which moves the body forward). Note: All joints are moved relative to their current position using the subroutine called inside the loop (shown in Figure 11).
sub FromCurServoPos(Port,Pos) // a + Pos is forward
if Port<=6
tcpc_send(char(0xAC+Port-1))
tcpc_send(char(CUR[Port]+Pos))
CUR[Port] = CUR[Port]+Pos
else
tcpc_send(char(0xAC+Port-1))
tcpc_send(char(CUR[Port]-Pos))
CUR[Port] = CUR[Port]-Pos
endif
return
FIGURE 11.
The subroutine FromCurServoPos can move any servo forward or backward a specified amount relative to its current position.
This routine also updates an array that keeps track of the current position of each servo. The actual servo movement is performed using TCP commands.
The amount to be moved can be either positive (moving the joint forward) or negative (moving the joint backward). Since servos on the right and left sides of the robot see forward and backward as opposite situations, the subroutine uses an IF statement to reverse the direction of movement as needed.
The sample code in Figures 10 and 11 is just a tiny portion of a demonstration program that allows the robot in Figure 1 to walk in a straight line over a level surface. Future modifications (such as altering the step routine that only lowers the leg until it reaches the ground, and using the accelerometer to keep the body tilted appropriately with respect to level rather than the ground) should allow the robot to handle hills and uneven terrain.
The video of the robot below is my first attempt at creating walking by utilizing a collection of routines that perform simple actions, such as shortening a leg or moving the body forward (as discussed earlier).
Even though the robot can walk, there are some things that need improving.
In the current program, when the robot wants to step with one leg, it shifts its weight away from that leg by shortening the opposing leg (and moving the tail).
This works fine when shortening a front leg, but when a rear leg is shortened, the robot’s weight shifts to the rear causing a slight backward movement.
It would be better if the robot’s momentum was always forward because these slight forward and backward shifts make the walking appear very unsteady as shown in the video.
Once you get a program like this working, you can fine-tune your subroutines to not only make your robot walk better, but to increase your understanding of the walking process as well.
There are many things left to experiment with, but that’s what hobby robotics is all about.
You could start by smoothing the robot’s walk, then make your robot utilize sensors to avoid objects by walking around them. Teach it how to climb a hill or maintain its balance in a cluttered environment.
I know these are all things I will enjoy experimenting with. Use my platform to get started or just use it for ideas and create your own system using a language of your choosing.
Hopefully, the accounts of my experiments with a walking robot will be helpful and motivational for anyone wanting to experiment with this topic.
The full source code for my current walking program can be downloaded from the IN THE NEWS tab at www.RoboBASIC.org for anyone wanting to study it. SV
Article Comments